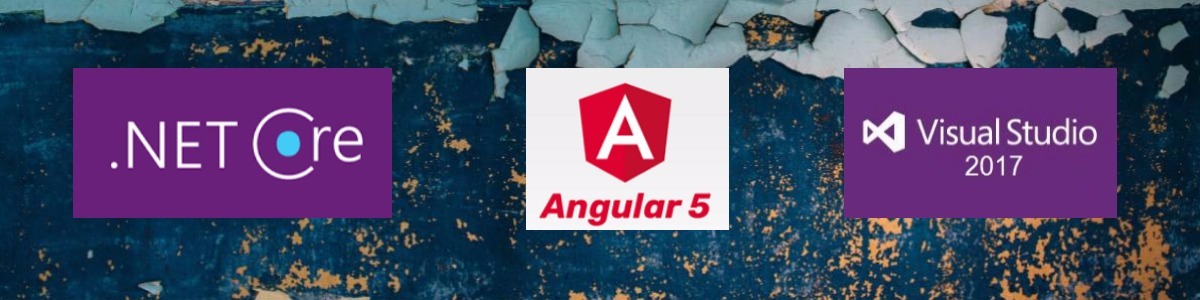
Angular 5 has been announced recently and we already have a template added in the Visual Studio 2017 with .Net Core 2.0
If you want to know more about Angular in .Net Core then my post may help you to get the basic which you can find here and if you want to see how can we deploy the Angular applications on Azure then have a look at my post here.
In this post, we will see how to add Angular 5 template in your Visual Studio and create an Angular 5 application using .Net Core 2.0 templates.
Prerequisite:
- Visual studio 2017 community edition, download here
- .Net Core 2.0 SDK from here (I have written a post to install SDK here)
- Node.js Version 9 or above from here
Add Angular 5 template
Once you have all these installed, open your Visual Studio 2017 -> Create New Project -> Go to Online tab and search with Angular5: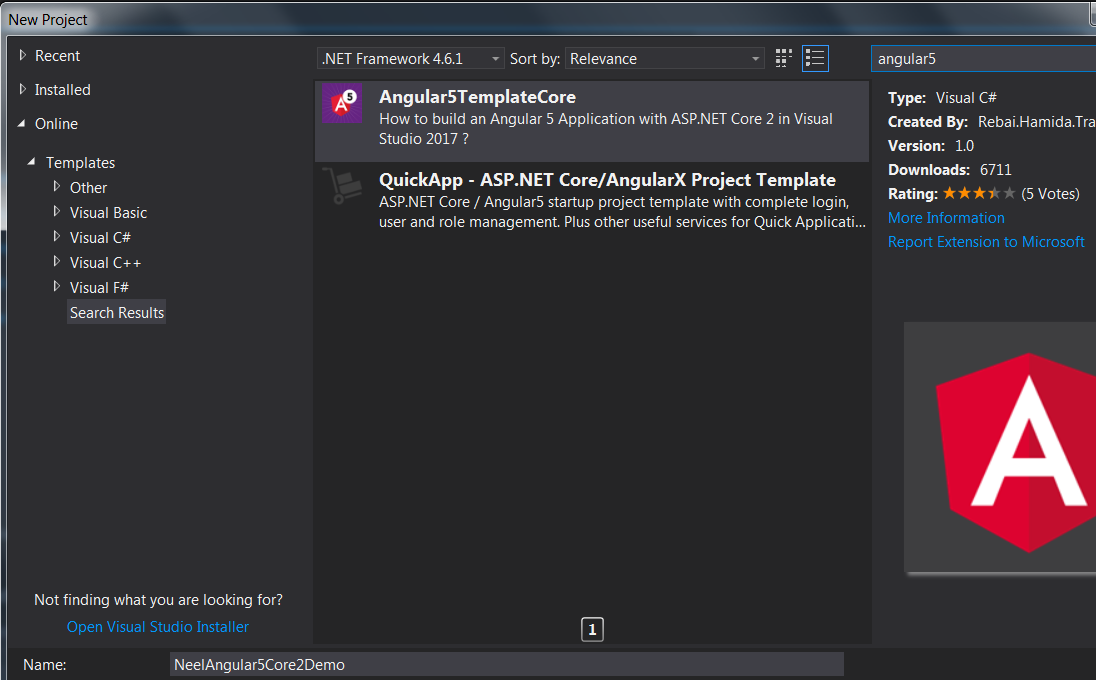
Select the Angular5TemplateCore and click on Ok.
Downloading of the template will be started once you click on Ok:
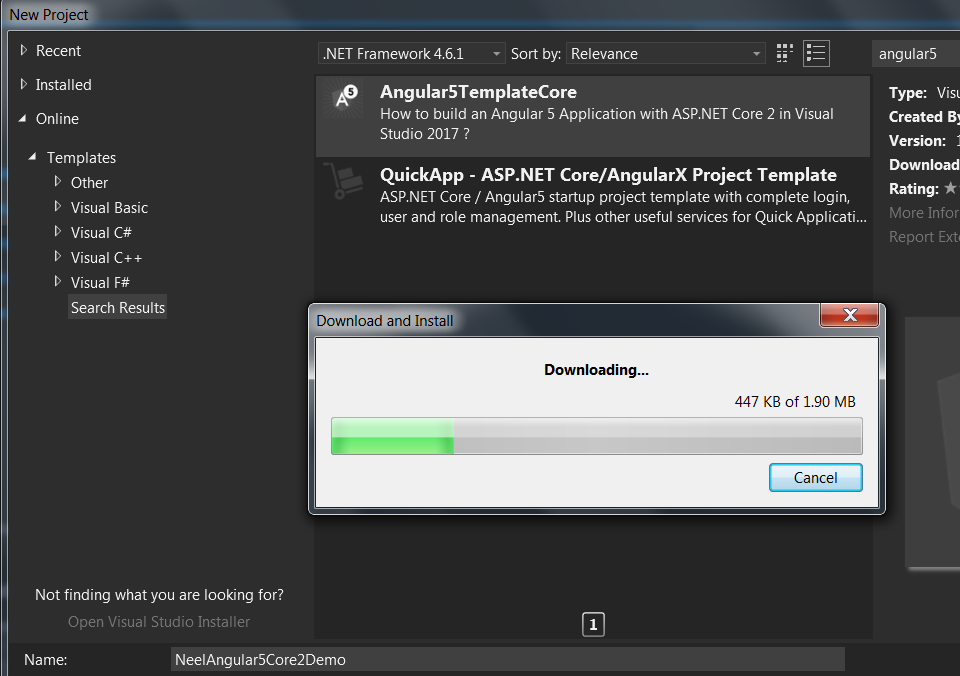
Once the download gets completed, an installer will be opened which will install the template in Visual Studio 2017.
Note - Close all running instances of the Visual Studio to have a smooth installation of the template.
The wizard will ask you to click on Modify:
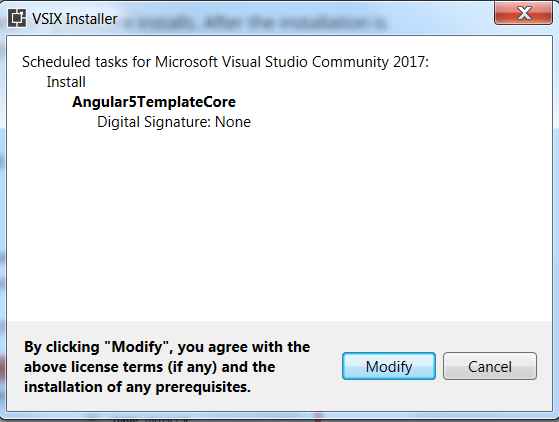
Once you click on Modify, the installation will be completed:

Create Angular 5 application
Once you have the template installed, open your Visual Studio 2017 -> Create New Project -> Select Installed tab and search with Angular5, now we would be able to see Angular5TF template:
Click on OK, Visual Studio will create a well-structured Angular 5 application for you:
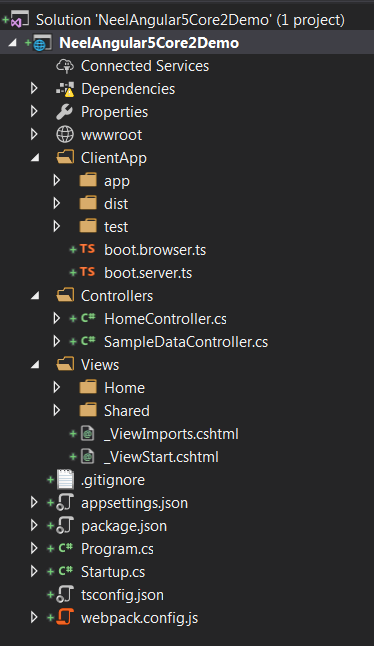
Now let us make sure that the application is Angular 5 application.
For that open package.json, here you will be able to see angular version 5.0.0 and Angular CLI version 1.5:
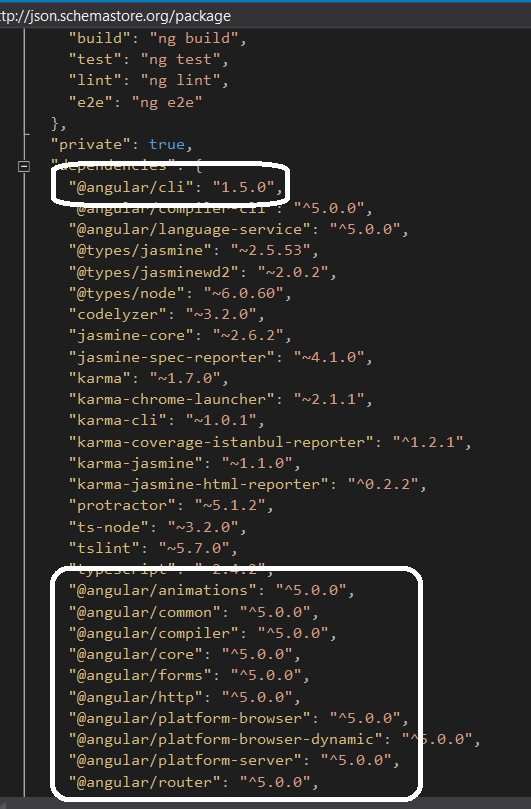
Next step is to install WebPack.
What is WebPack?
WebPack is a module bundler.WebPack is used to determine the dependencies, run transformations and create bundles that you can provide to your browser.
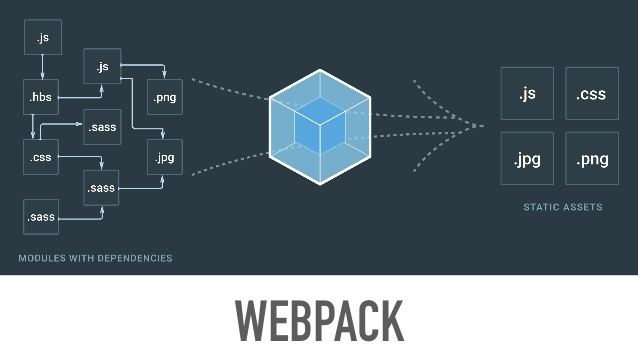
It is a good practice to add WebPack to the starting point that is why we will add below lines in package.json file if it is not there already:
"postinstall": "webpack --config webpack.config.vendor.js"
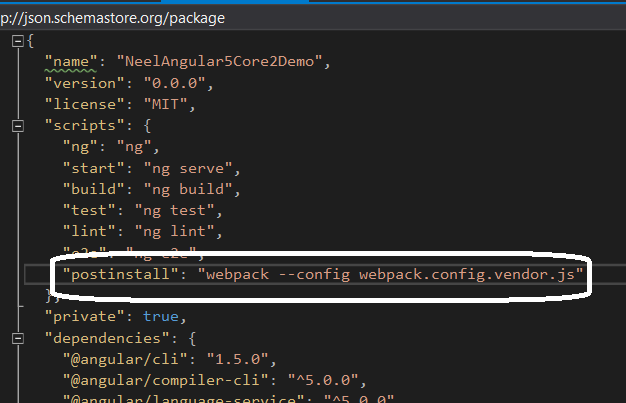
You may get below error if you have not added WebPack details in the package.json file:
Cannot find module './wwwroot/dist/vendor-manifest.json'
To avoid this error, we need to run above WebPack command first. That is why we have added the command to the package.json file.After adding above line, build the project. First time when you build the solution, it would take several minutes as it installs some dependencies.
Run the Angular application
Now click on IISExpress to run the application.After 5-10 seconds, the application might have compilation error as below in Vendor.js file:
An exception has been encountered. This may be caused by an extension.
You can get more information by examining the file 'C:\Users\neel\AppData\Roaming\Microsoft\VisualStudio\15.0_b495e641\ActivityLog.xml'.
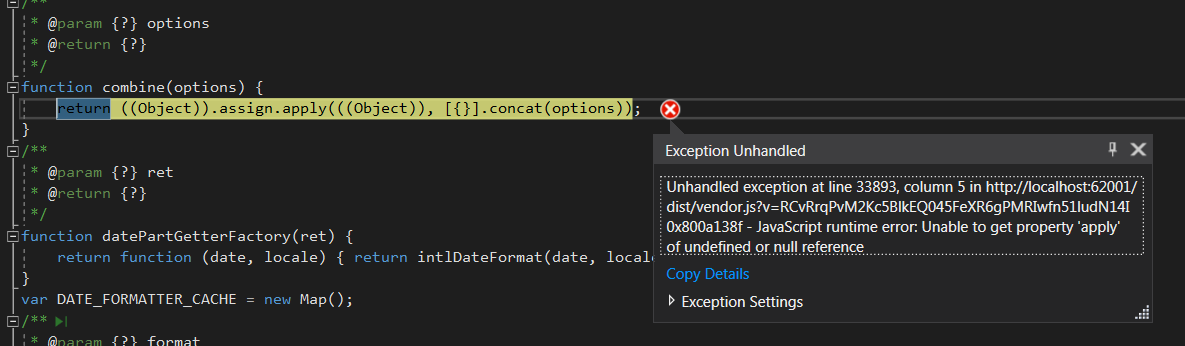
This error comes because IE does not support several functions used in the project. You need to import some libraries to resolve this error. Have a look here to resolve the error.
Once the error is resolved, the application will run properly:

Hope it helps.